51일차
----------------------------
ATMega128 시리얼 통신
----------------------------
--- ATMega128 -> PC로 데이터 보내기
ex) 소스
- main.c
#include "smart.h"
#include "Lcd.h"
#include "Usart.h"
int main(void)
{
volatile unsigned int uiCnt;
// Initialization
LCD_Init();
Usart_Init();
LCD_Inst(INST_SET_DDRAM | 0x00);
LCD_Str("abcd");
LCD_Inst(INST_SET_DDRAM | 0x40);
LCD_Str("1234");
Usart_Str("Hello There~\r\n");
while(1);
return 0;
}
- Usart.h
#ifndef _USART_H_
#define _USART_H_
#include "smart.h"
//UART Reg
#define UDR0 (*((volatile unsigned char *)0x2C))
#define UCSR0A (*((volatile unsigned char *)0x2B))
#define UCSR0B (*((volatile unsigned char *)0x2A))
#define UCSR0C (*((volatile unsigned char *)0x95))
#define UBRR0L (*((volatile unsigned char *)0x29))
#define UBRR0H (*((volatile unsigned char *)0x90))
#define UDR1 (*((volatile unsigned char *)0x9C))
#define UCSR1A (*((volatile unsigned char *)0x9B))
#define UCSR1B (*((volatile unsigned char *)0x9A))
#define UCSR1C (*((volatile unsigned char *)0x9D))
#define UBRR1L (*((volatile unsigned char *)0x99))
#define UBRR1H (*((volatile unsigned char *)0x98))
#define BAUD 57600 // bps speed
#define UBRR (((F_OSC)/((BAUD)*16L)) - 1) // 공식
//UCSRA Reg each bit location
#define RXC 7
#define TXC 6
#define UDRE 5
#define FE 4
#define DOR 3
#define UPE 2
#define U2X 1
#define MPCM 0
//UCSRB Reg each bit location
#define RXCIE 7
#define TXCIE 6
#define UDRIE 5
#define RXEN 4
#define TXEN 3
#define UCSZ2 2
#define RXB8 1
#define TXB8 0
//UCSRC Reg each bit location
#define UMSEL 6
#define UPM1 5
#define UPM0 4
#define USBS 3
#define UCSZ1 2
#define UCSZ0 1
#define UCPOL 0
void Usart_Init(void);
void Usart_Tx(const char cNum);
void Usart_Str(const unsigned char * cString);
unsigned char Usart_Rx(void);
#endif // _USART_H_
- Usart.c
#include "Usart.h"
void Usart_Init(void)
{
// setting speed
UBRR0H = UBRR>>8;
UBRR0L = UBRR;
// setting Mode
UCSR0A = (0<<TXC) | (0<<U2X) | (0<<MPCM);
UCSR0B = (0<<RXCIE) | (0<<TXCIE) | (0<<UDRIE) |
(1<<RXEN) | (1<<TXEN) | (0<<UCSZ2);
UCSR0C = (0<<UMSEL) | (1<<UPM1) | (0<<UPM0) |
(0<<USBS) | (1<<UCSZ1) |(1<<UCSZ0);
}
void Usart_Tx(const char cNum)
{
while(0 == (UCSR0A & (1<<TXC)));
UDR0 = cNum;
}
void Usart_Str(const unsigned char * cString)
{
while(0 != *cString)
{
Usart_Tx(*cString);
++cString;
}
}
unsigned char Usart_Rx(void)
{
while(0 == (UCSR0A & (1<<RXC)));
return UDR0;
}
출력 화면
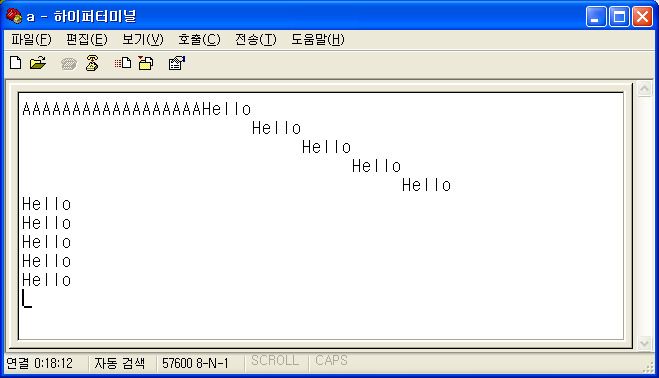
계속 통신을 시험했을 때 오류가 났었는데
ATMega128로 프로그램을 넣을 때 변환해 주는 칩을 낀 상태로 실행을 하니
제대로 실행이 안됐었다.
알고 보니 그 칩에도 TxD0, RxD0가 연결되어 보내는 신호가 잘 안됐었다.
- UBRR (((F_OSC)/((BAUD)*16L))-1)
여기서 L을 붙인 이유는 ATMega128은 8bit이기 때문에 2byte가 넘는 수는
계산할 수 없다. 그래서 4byte 데이터인 Long 형으로 캐스팅 해준다는 뜻.
- Usart_Tx(), Usart_Rx() 함수를 보면
현재 송신이 끝났는지, 수신이 끝났는지 확인(while문)하고 데이터를 사용한다.
- C
-----------------
파일 읽기 쓰기
-----------------
- fread, fwrite
원형
size_t fread(void * buf, size_t size, size_t count, FILE * stream);
size_t fwrite(const void * buf, size_t size, size_t count, FILE * stream);
성공시 전달인자 count, 실패 또는 파일 끝 도달시 count보다 작은 값 반환.
buf는 말 그대로 메모리 공간(정보 저장된 곳 or 정보 저장할 곳)
size, count는 서로 곱해서 buf 크기 만큼 나오면 된다.
stream은 쓰거나 읽을 파일 스트림을 말한다.
ex) 소스
#include <stdio.h>
typedef struct _smart
{
int iNum;
char a;
int iNum2;
char b;
short c;
}Smart;
int main()
{
FILE * fp;
Smart test;
Smart test2;
printf("%d \n", sizeof(Smart) );
fp = fopen("AA.bin", "wb");
test.iNum = 0x41424344;
test.a = 'Z';
test.iNum2 = 0x45464748;
test.b = 'Y';
test.c = 0x494A;
fwrite(&test, sizeof(Smart), 1, fp);
fclose(fp);
fp = fopen("AA.bin", "rb");
fread(&test2, sizeof(Smart), 1, fp);
printf("%08X \n", test2.iNum);
printf("%c \n", test2.a);
printf("%08X \n", test2.iNum2);
printf("%c \n", test2.b);
printf("%04X \n", test2.c);
fclose(fp);
return 0;
}
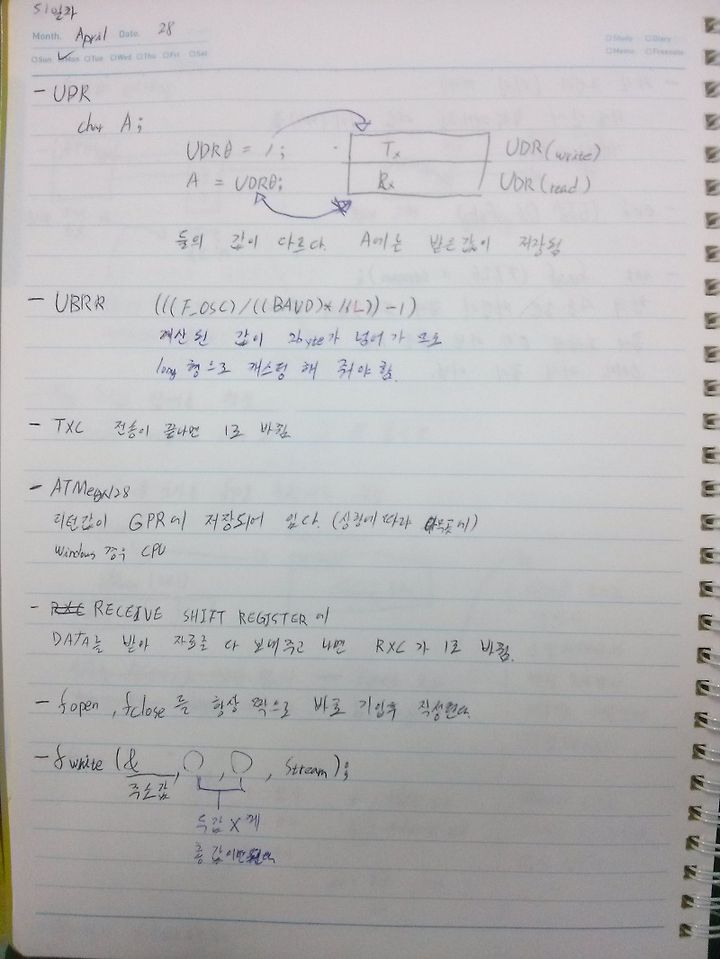